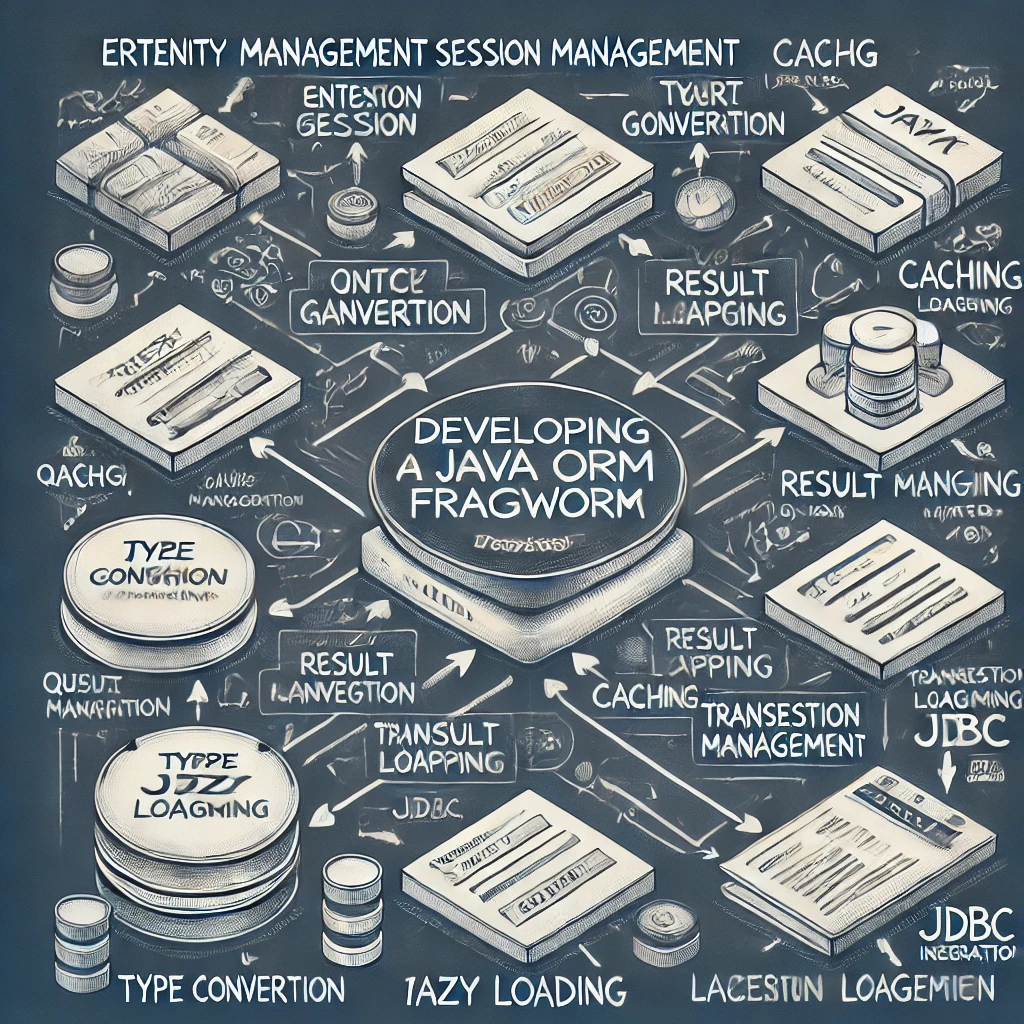
Developing a full-fledged ORM framework that can rival Hibernate requires careful planning and attention to both design and implementation. It will involve creating an abstraction layer that manages database interactions, much like Hibernate, while supporting features like automatic entity mapping, caching, query generation, and more.
Here’s a blueprint for developing an ORM framework that could become a remarkable alternative to Hibernate:
Blueprint for Developing a Java ORM Framework
1. Core Design Considerations
Before writing any code, define your framework’s core goals and key features:
- Database Independence: The ORM framework should support multiple databases with minimal configuration.
- Ease of Use: Provide a user-friendly API that minimizes the effort required for developers.
- Performance: Optimize SQL queries and reduce overhead.
- Extensibility: Ensure that the framework is extensible so that you can add new features over time.
- Transactions: Implement robust transaction management (auto-commit, commit, rollback).
- Query Generation: Dynamically generate SQL queries based on entities and relationships (no need for manual SQL).
- Caching: Implement caching for performance improvements.
- Lazy Loading: Support for lazy loading of relationships.
- Type Conversion: Proper mapping of Java types to database types.
2. Define Core Components
To develop an ORM like Hibernate, we need to structure the framework into distinct modules. Here’s a breakdown of the key components:
a. Entity Management
Entities represent Java objects that map to database tables. They should support annotations or XML configurations for mapping.
- Annotations (like
@Table
,@Column
,@Id
) will be used to define mappings between classes and database tables, fields and columns, etc.
javaCopy code@Table(name = "students")
public class Student {
@Id
private int id;
@Column(name = "name")
private String name;
@Column(name = "age")
private int age;
// Getters and setters...
}
b. Session Management
Session management is crucial for managing database connections and transactions in your ORM framework. The Session
will serve as the interface between your application and the database.
- The
Session
should handle:- Opening and closing connections.
- Beginning, committing, and rolling back transactions.
- Executing queries (both standard and HQL/JPQL-style queries).
javaCopy codepublic class Session {
private Connection connection;
private boolean inTransaction;
public void beginTransaction() { /* Code to begin transaction */ }
public void commit() { /* Code to commit transaction */ }
public void rollback() { /* Code to rollback transaction */ }
public Connection getConnection() { /* Get connection */ }
}
c. Entity Manager
The EntityManager
will handle CRUD operations for entities. It provides methods to persist, merge, remove, and find entities.
javaCopy codepublic class EntityManager {
public <T> void persist(T entity) {
// Save entity to the database
}
public <T> T find(Class<T> entityClass, Object primaryKey) {
// Retrieve an entity from the database by its primary key
return entity;
}
public <T> void remove(T entity) {
// Delete an entity from the database
}
}
d. Query Generation
Your framework should be able to dynamically generate SQL queries based on the annotations in your entities or HQL/JPQL-style queries.
- SQL Generation: The framework should be able to create
SELECT
,INSERT
,UPDATE
, andDELETE
queries based on entity properties.
javaCopy codepublic class QueryBuilder {
public String generateSelectQuery(Class<?> entityClass) {
// Generates a SELECT query based on annotations or entity field mappings.
return "SELECT * FROM " + entityClass.getSimpleName();
}
public String generateInsertQuery(Object entity) {
// Generates an INSERT query based on entity fields
}
}
e. Type Conversion and Result Mapping
- Type Conversion: Java types (e.g.,
String
,Integer
) should be mapped to the appropriate database types (e.g.,VARCHAR
,INT
).
javaCopy codepublic class TypeConverter {
public static Object convertToDatabaseType(Object javaValue) {
// Convert Java object to database-compatible value (e.g., Date, Integer).
}
public static Object convertFromDatabaseType(Object dbValue, Class<?> targetType) {
// Convert database value to Java object (e.g., Integer to Integer).
}
}
- Result Mapping: When retrieving records, you’ll need to convert
ResultSet
data to entity objects.
javaCopy codepublic class ResultMapper {
public <T> T mapResultSetToEntity(ResultSet rs, Class<T> clazz) {
// Map ResultSet row data to the entity class
}
}
3. Additional Features for Your ORM Framework
a. Transaction Management
Transactions are a key feature for managing consistency and atomicity in database operations.
- Implement transaction management within the session or entity manager.
- Implement
commit()
,rollback()
, andsavepoint
functionality.
b. Lazy and Eager Loading of Relationships
- Implement lazy loading for
OneToMany
orManyToOne
relationships so that associated objects are loaded only when accessed. - Eager loading should load related entities when the main entity is loaded.
Example of Lazy Loading:
javaCopy code@Entity
public class Student {
@ManyToOne(fetch = FetchType.LAZY)
private Course course;
}
c. Caching
Implement caching for entities to reduce the number of database queries. Use a first-level cache (per session) and a second-level cache (shared across sessions).
javaCopy codepublic class CacheManager {
private Map<String, Object> cache;
public void put(String key, Object value) {
cache.put(key, value);
}
public Object get(String key) {
return cache.get(key);
}
}
d. Automatic Schema Generation
Allow automatic schema creation and updates based on your entity classes, much like Hibernate’s Hibernate Tools.
- You can inspect annotations and auto-generate SQL
CREATE TABLE
andALTER TABLE
commands.
e. Query Language (Optional)
If you want your framework to compete with Hibernate, consider creating your own query language that’s similar to HQL or JPQL (Java Persistence Query Language), which abstracts SQL.
javaCopy codepublic class QueryParser {
public String parse(String query) {
// Parse the user-defined query (like HQL or JPQL) into a SQL query.
}
}
4. Integration with JDBC
Since this ORM framework will not use external libraries like Hibernate or JPA, it will rely on JDBC for database communication. Create helper classes for JDBC connection pooling, statement execution, and result set processing.
javaCopy codepublic class JDBCHelper {
public static Connection getConnection() {
// Get a connection to the database
}
public static void closeConnection(Connection conn) {
// Close the connection
}
}
5. Extensibility and Configurations
- Property Files: Use property files or annotations to configure the framework (e.g., database connection details, transaction isolation level).
- Plugin Support: Allow plugins to add new functionality, such as custom query builders or database-specific optimizations.
- Support for Multiple Databases: Design your framework to be flexible enough to support various databases like MySQL, PostgreSQL, Oracle, etc.
6. Testing and Benchmarking
Before releasing your ORM framework:
- Unit Tests: Write comprehensive unit tests for all core components.
- Integration Tests: Test integration with actual databases.
- Performance Benchmarks: Benchmark the ORM’s performance and compare it to Hibernate for certain use cases (e.g., data fetching, batch processing).
7. Documentation and API Design
A well-designed API and thorough documentation are crucial for adoption.
- Provide detailed documentation on how to use your ORM.
- Use JavaDocs for all public methods and classes.
- Provide examples for common use cases.
Final Thoughts
Building your own ORM is a challenging but highly rewarding project. It will involve deep knowledge of database operations, reflection, annotation processing, and design patterns. Aim to start small and gradually add features (e.g., start with basic CRUD operations, then expand to advanced features like caching and lazy loading).
While Hibernate and JPA are industry standards, a custom ORM framework can be tailored to your specific needs, potentially offering performance improvements or other specialized features.